1. C# APIs¶
MetaSensors are supported on Windows. Developers can create their own Apps using C# on Windows.
For this tutorial, you should be comfortable with Windows, the terminal (command-prompt), C#, Visual Studio and dotnet.
We will be using Visual Studio and the terminal.
Note
Windows 10 or newer is required. BluetoothLE is no supported on Windows 8 or older.
1.1. Installation¶
Ensure that you are running Windows 10 or newer (Windows 7 or older is not supported):
- Select the Start button, then select Settings > System > About.
- Under Windows specifications, you can find out which edition and version of Windows your device is running. It must be a version of Windows 10 or higher.
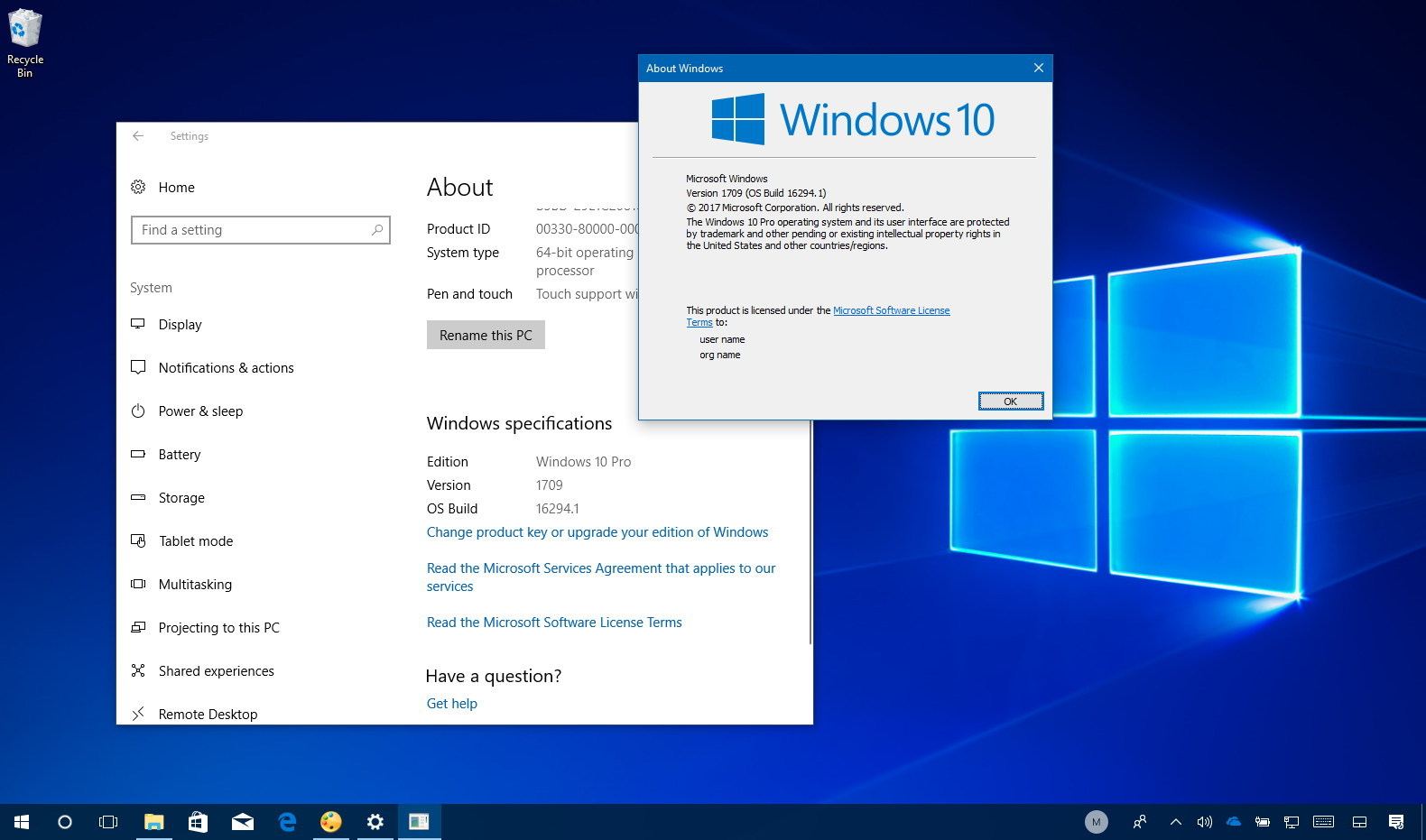
- You must be at least on the Fall Creator’s Update of Windows 10. Once you’re in the About page, on “Version”, you should see the 1709 number, and under “OS Build”, the number should be 16299.192 or later.
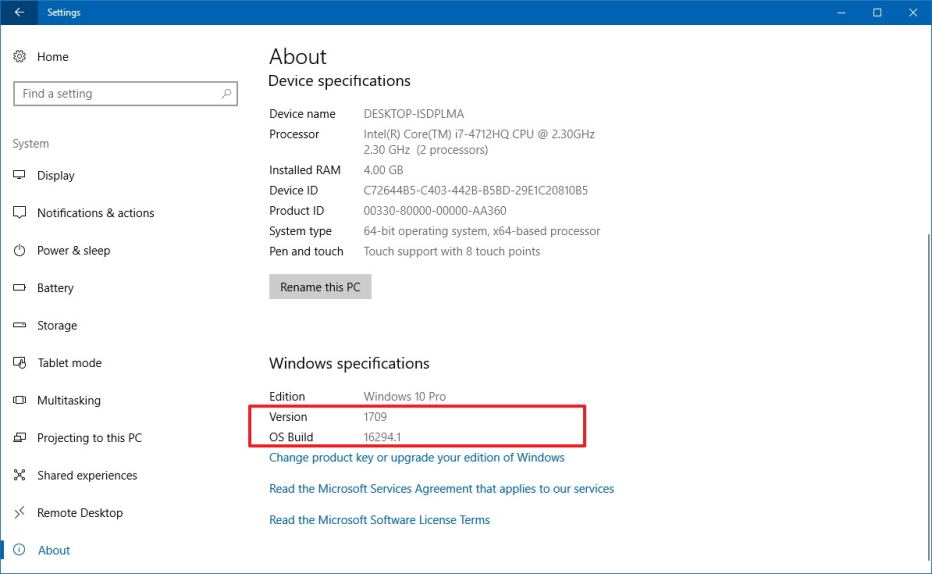
1.1.1. Bluetooth¶
Your computer needs to support Bluetooth Low Energy (Bluetooth 4.0 or later) to work with MetaSensors. First you will need to check if your computer supports it as-is by following the steps below:
- Open Start.
- Search for Device Manager and click the top result.
- Expand the Bluetooth category.
- Right-click the Bluetooth adapter, and select Properties.
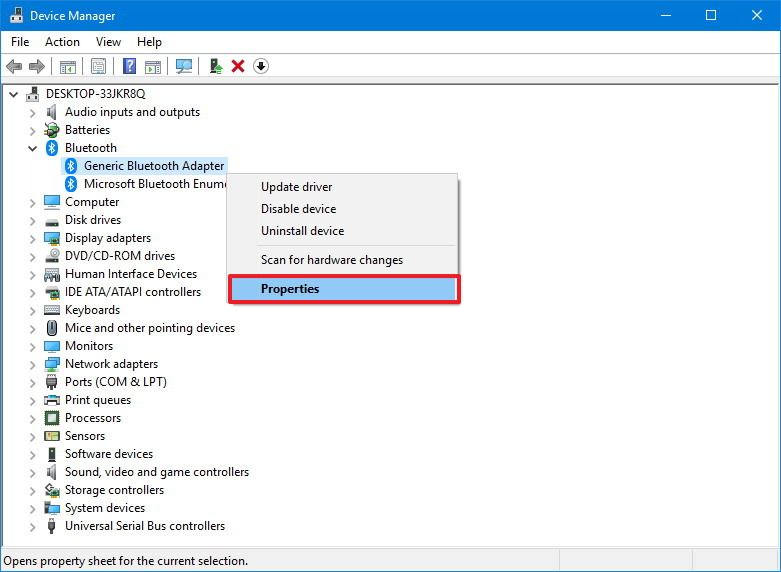
- Click on the Advanced tab.
- Under “Firmware,” make a note of the LMP version number.
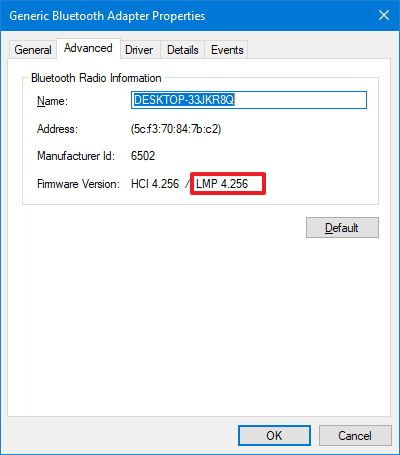
- After completing the steps, check the LMP number using the list below to find out the actual Bluetooth version:
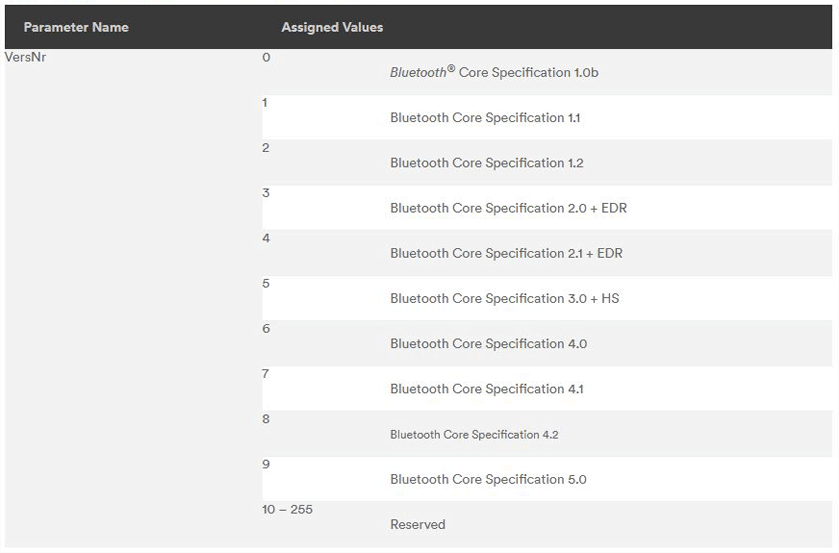
- If your computer does not support Bluetooth 4 (LMP 6) or higher you will need a Bluetooth Dongle. You can purchase a BLE Dongle on our store.
- Plug in the dongle into a USB slot on your PC.
- Automatically install the Bluetooth drivers. Windows 10 includes the basic Broadcom Bluetooth drivers required by the dongle and will install them automatically when it recognizes the new device.
Note
Windows 10 only supports 1 USB to BLUETOOTH dongle.
Because Bluetooth is limited on Windows and only 1 dongle is supported, we recommend that only 3 devices be connected simultaneously. You can always connect to 3 devices, access sensor data, disconnect and then connect to 3 other devices in a round-robin fashion for optimal performance.
1.1.2. Visual Studio¶
Download the Visual Studio 2017 Installer here.
Note
We do not support VS2018, VS2019, or VS2020. You can have multiple instalations however our APIs ONLY work with VS2017.
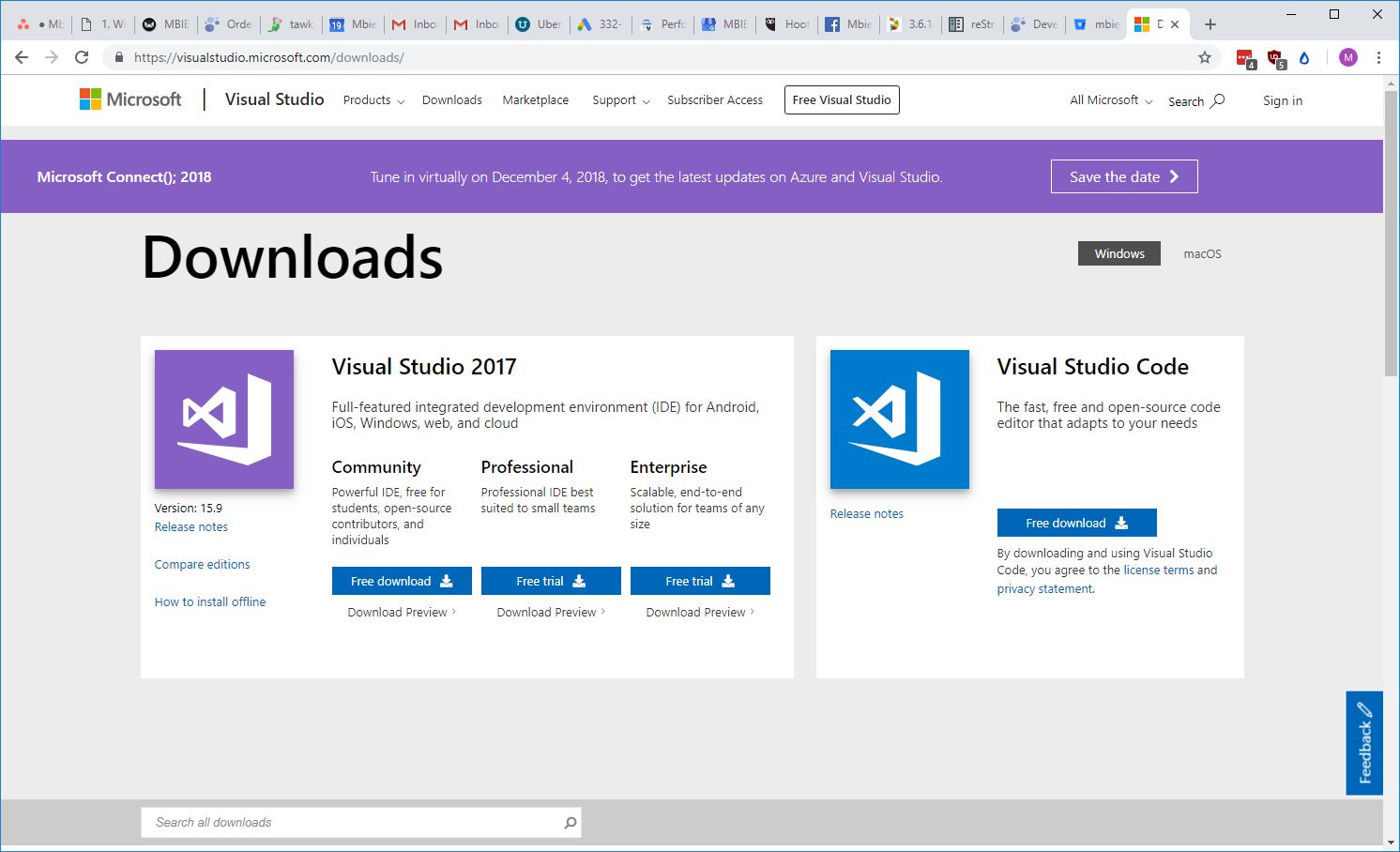
You can select:
- Visual Studio 2017 Community Edition
- Visual Studio 2017 Professional Edition (30 Day Free Trial)
Warning
MetaWear APIs require VS2017. YOU MUST USE VISUAL STUDIO 2017!
If you have another Visual Studio version on your machine, please install the 2017 edition.
Click on the downloaded .exe file. Open up Visual Studio Installer.
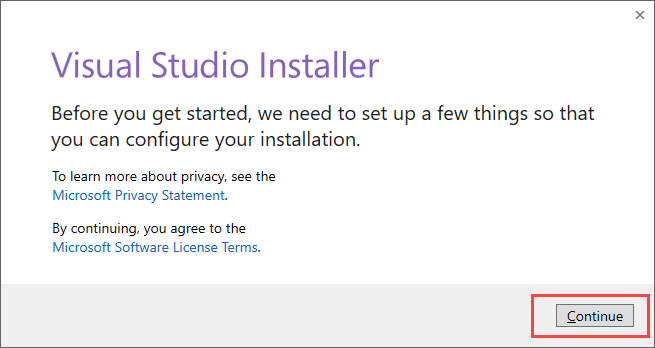
You will be prompted to create or use your existing Microsoft login.
Visual Studio will start downloading the initial files. Download speed will vary as per your internet connection.
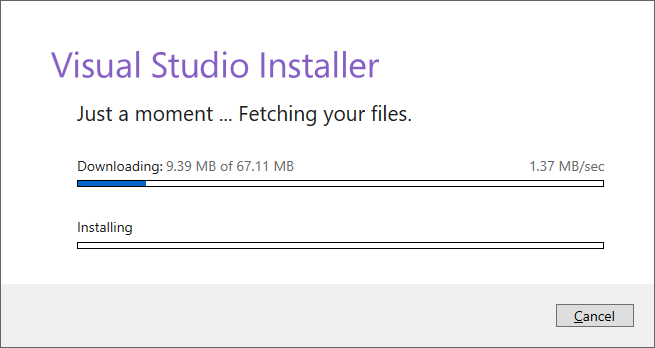
Install Visual Studio. Click Install on the appropriate Product section.
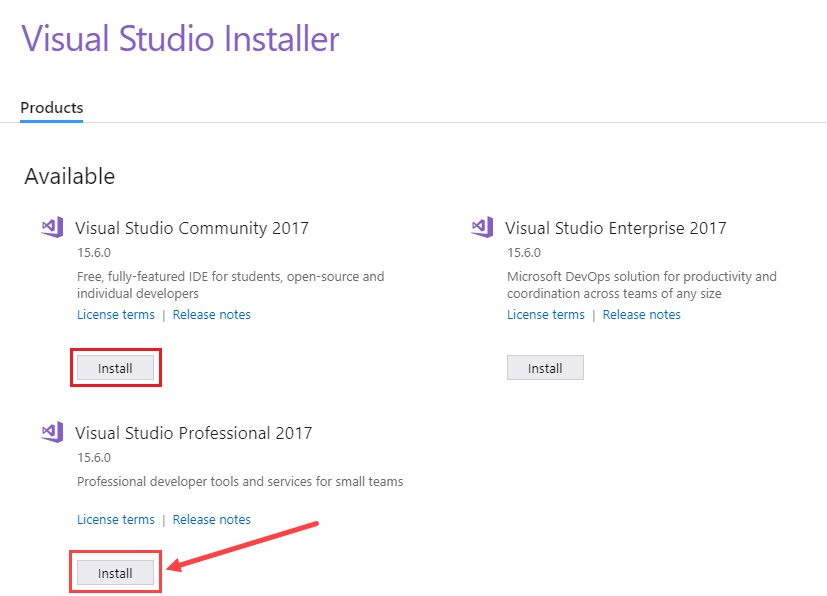
Install all of the required individual components by selecting “Individual components”.
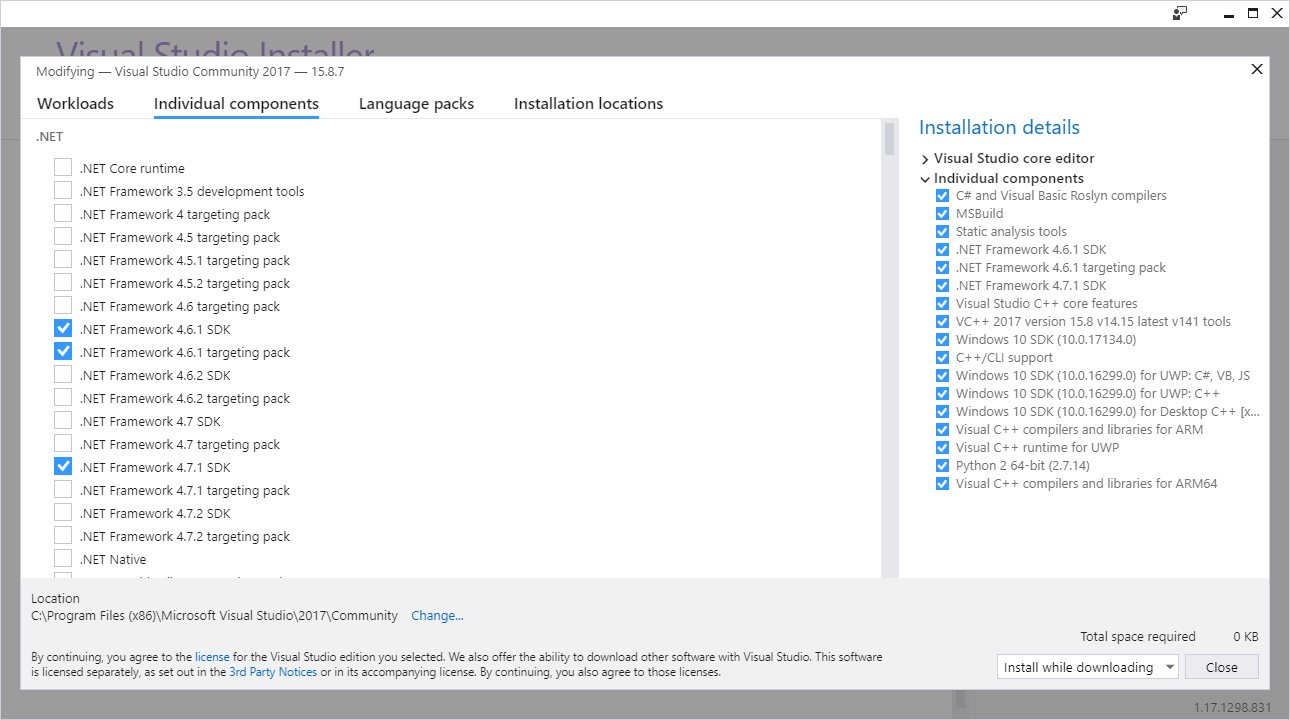
You must install:
- C# and Visual Basic Roslyn compilers
- MSBuild
- Static analysis tools
- .NET Framework 4.6.1 SDK
- .NET Framework 4.6.1 targeting pack
- .NET Framework 4.7.1 SDK
- Visual Studio C++ core features
- VC++ 2017 version 15.8 v14.15 latest v141 tools
- Windows 10 SDK (10.0.17134.0)
- C++/CLI support
- Windows 10 SDK (10.0.16299.0) for UWP: C#, VB, JS
- Windows 10 SDK (10.0.16299.0) for UWP: C++
- Windows 10 SDK (10.0.16299.0) for Desktop C++ [x86 and x64]
- Visual C++ compilers and libraries for ARM
- Visual C++ runtime for UWP
- Visual C++ compilers and libraries for ARM64
Visual Studio Installer will download the relevant files based on all of your selections.
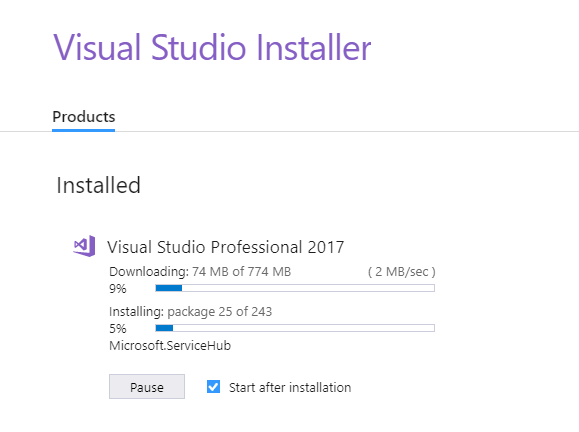
You may need to reboot your computer.
If you are new to visual studio, please follow the Microsoft tutorials:
1.1.3. Environment¶
To enable MSBUILD in the Command Prompt, you have to add the path to the .NET 4 Framework and Python executables on your machine to the PATH environment variable.
You can access the environment variables by:
- Right clicking on Computer
- Click Properties
- Then click Advanced system settings on the left navigation bar
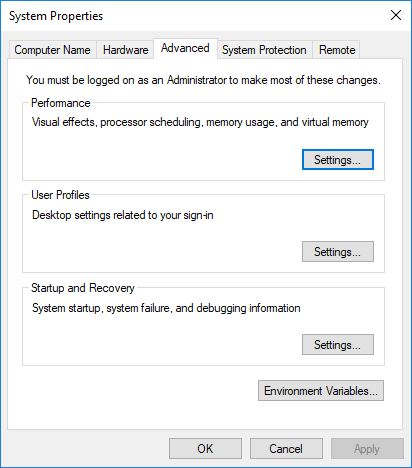
- On the next dialog box click Environment variables
- Scroll down to PATH
- Edit it to include your MSBUILD and Python (including the Scripts subfolder) paths to the framework (don’t forget a “;” after the last entry in here or add them one at a time).
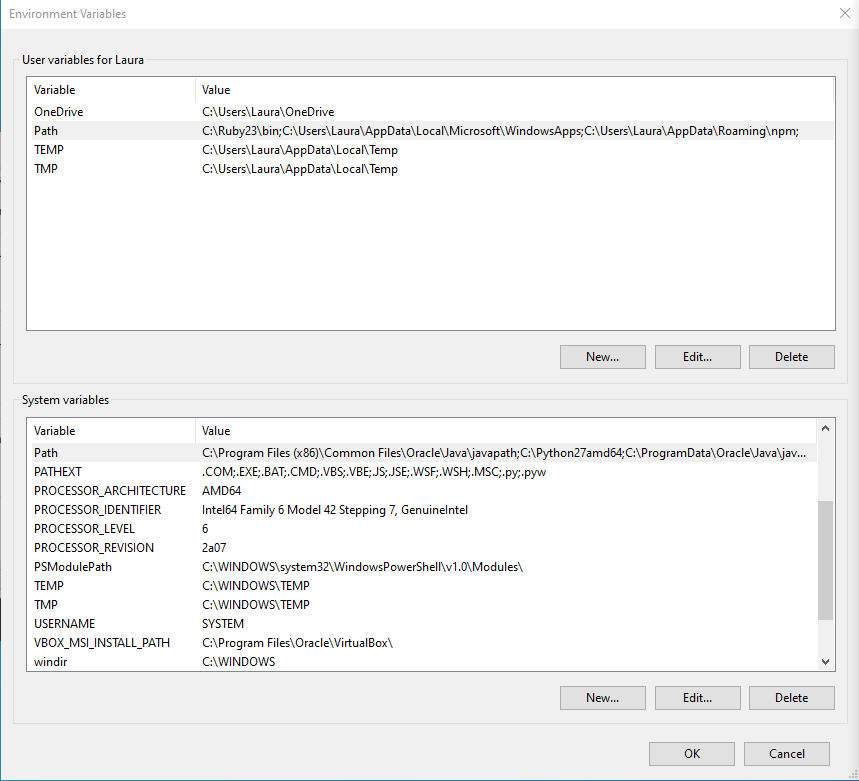
I added the MSBUILD path to my environmental variables. Here is another one of my environment variables setup for your reference:

A good final check is to make sure that platform.winmd
is installed in the proper location on your machine:
- C:\Program Files (x86)/Microsoft Visual Studio/2017/Community/VC/Tools/MSVC/14.15.26726/lib/x86/store/references
- C:\Program Files (x86)/Microsoft Visual Studio/2017/Community/Common7/IDE/VC/vcpackages
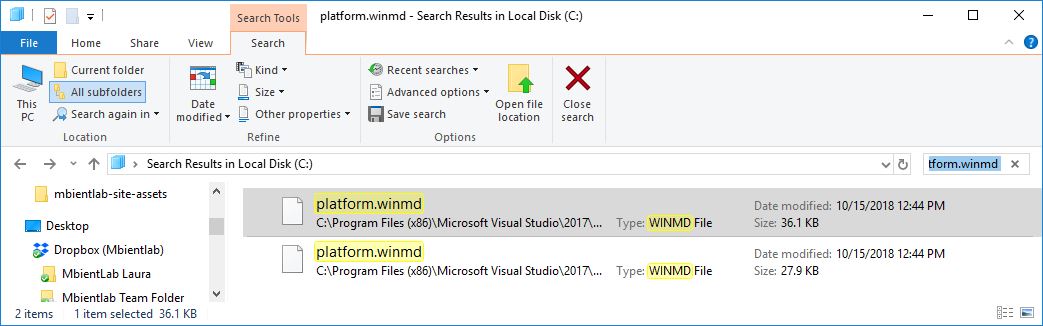
1.2. Dependencies¶
1.2.1. Nuget¶
NuGet is the package manager for .NET. The NuGet client tools provide the ability to produce and consume packages.
Nuget is automatically installed with Visual Studio 2017.
The MetaWear Nuget package can be found here. We will install it in the steps below.
1.2.2. DotNet¶
The C# SDK can be used to create both Universal Windows Platform and .NET Core applications on Windows 10.
Depending on which type of application you are building, the setup steps will be slightly different.
This tutorial will cover how to use the the SDK with .NET Core console applications.
You will need to install the .NET Core toolset from the Workloads page.
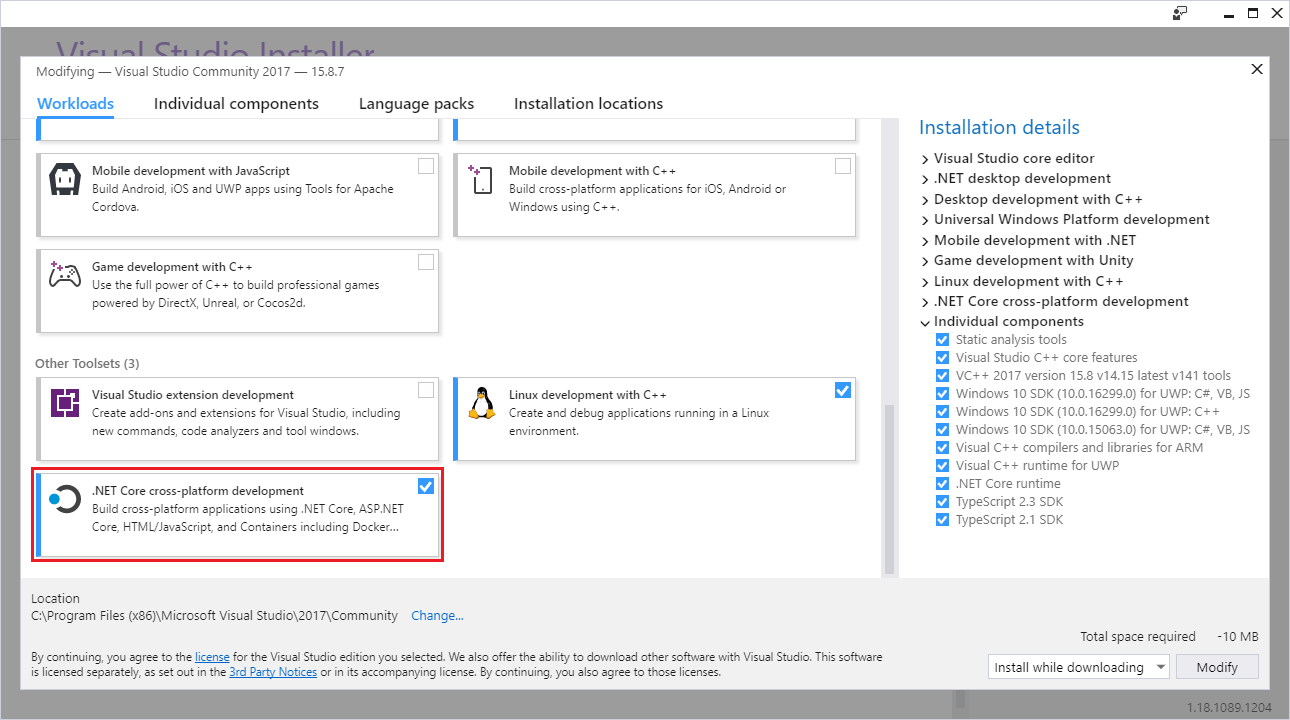
Double check that you have properly installed .NET Core by printing the installed version:
>>> dotnet --version

If you want to learn more, please follow this tutorial from Microsoft.
1.3. MetaWear¶
After you have confirmed that dotnet
is properly installed:
- Create a new console application called
NetCoreExamples
.
>>> dotnet new console -n NetCoreExamples
>>> cd NetCoreExamples
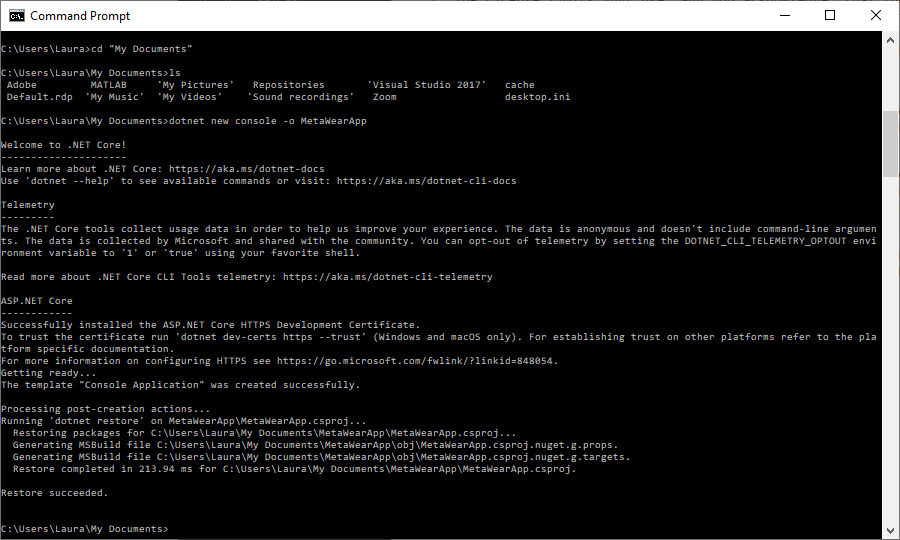
- Add a dependency to
MetaWear.CSharp
andMetaWear.CSharp.NetStandard
.
>>> dotnet add package MetaWear.CSharp
>>> dotnet add package MetaWear.CSharp.NetStandard
- Open
Program.cs
and replace the contents with:
using System;
using System.Linq;
using System.Reflection;
using System.Threading.Tasks;
namespace NetCoreExamples {
class Program {
static void Main(string[] args) {
MainAsync(args).Wait();
}
private static async Task MainAsync(string[] args) {
var type = Type.GetType(args[0]);
await (Task)type.GetMethod("RunAsync", BindingFlags.NonPublic | BindingFlags.Static)
.Invoke(null, new object[] { args.TakeLast(args.Length - 1).ToArray() });
}
}
}
After adding new code blocks to the project, build the project and fix any reported errors before continuing.
>>> dotnet build
1.4. Usage¶
The C# SDK is distributed via NuGet and can be installed with the Nuget package manager console:
>>> Install-Package MetaWear.CSharp
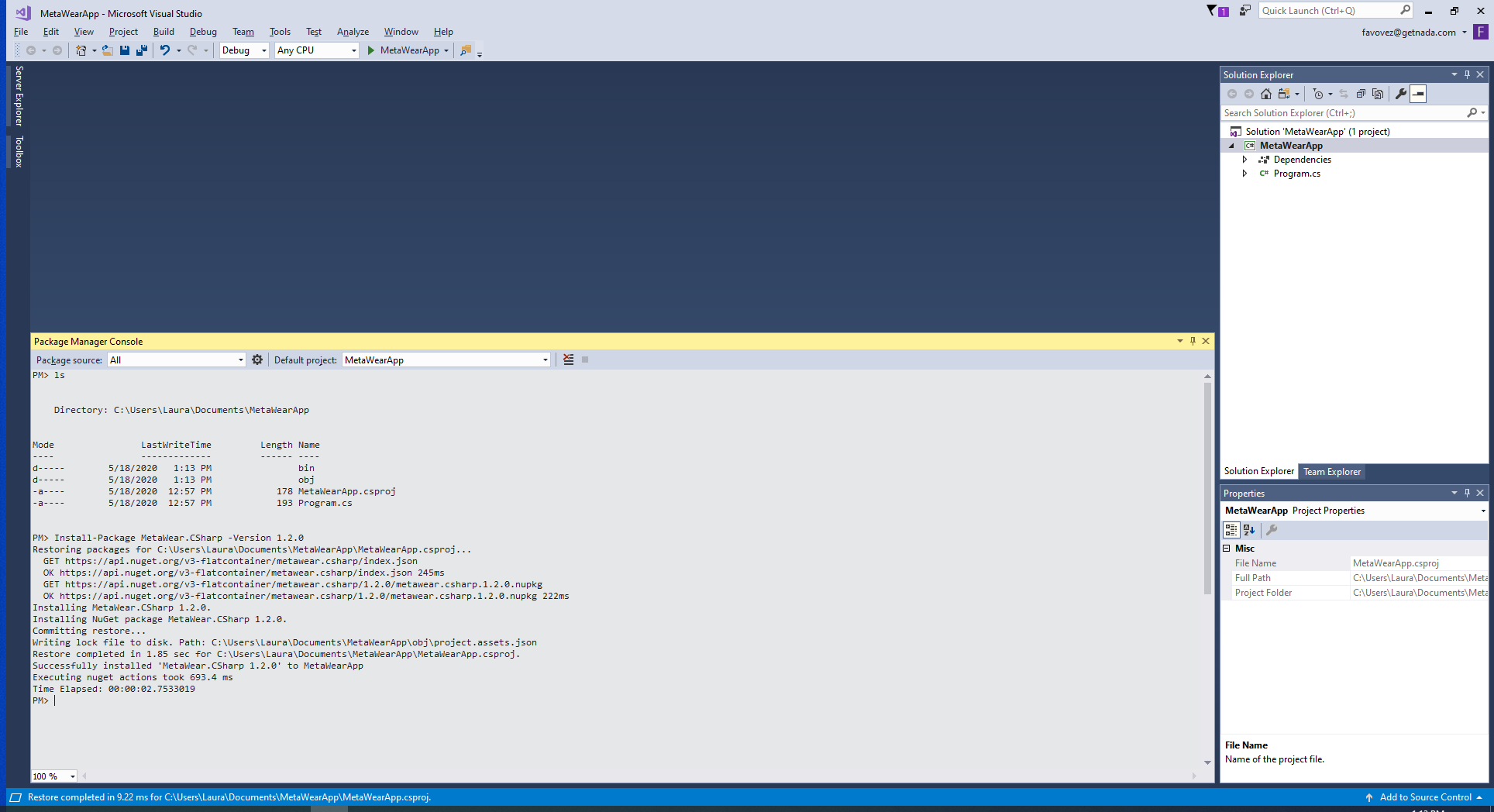
MbientLab provides an implementation of the MetaWear API in the Impl namespace
.
Similiarly to the top level interfaces, the main class in the Impl namespace
is the MetaWearBoard class.
To instantiate a MetaWearBoard object, you will need to provide an implementation of the IBluetoothLeGatt and the ILibraryIO interfaces:
using MbientLab.MetaWear.Impl.Platform;
class BluetoothLeGatt : IBluetoothLeGatt {
// Implementation here
}
class IO : ILibraryIO {
// Implementation here
}
var metawear = new MetaWearBoard(new BluetoothLeGatt(), new IO());
1.4.1. Download API Repository¶
Head over to our C# Github page: https://github.com/mbientlab/MetaWear-SDK-CSharp
You can clone the repository or simply download as a ZIP file:
>>> git clone https://github.com/mbientlab/MetaWear-SDK-CSharp
1.4.2. About C# APIs¶
The SDK is distributed on NuGet as a platform agnostic, .NET Standard 2.0 assembly.
Due to its platform agnostic nature, developers will need to plugin their own BLE stack and file i/o code specific to their target environment.
When you download our Repository, you will find:
- MetaWear -> The C# APi source code
- MetaWear.test -> Tests
- nuget -> nuget spec
- examples -> the source code for the examples further discussed below
In the examples directory, there are a couple of Windows applications to try out:
- BtleDeviceScanner
- FreeFall Detector
- NetCoreExamples
- RealTimeGraph
- StarterApp
We also have C# API documentation here. Please keep this documentation handy.
1.5. Sample Apps¶
The example directory is full of sample Apps. Here will will open and run the RealTimeGraph App.
- Open Visual Studio.
- Open the RealTimeGraph project in VS2017 (or open all the projects at once by opening
MetaWear Tutorials.sln
).
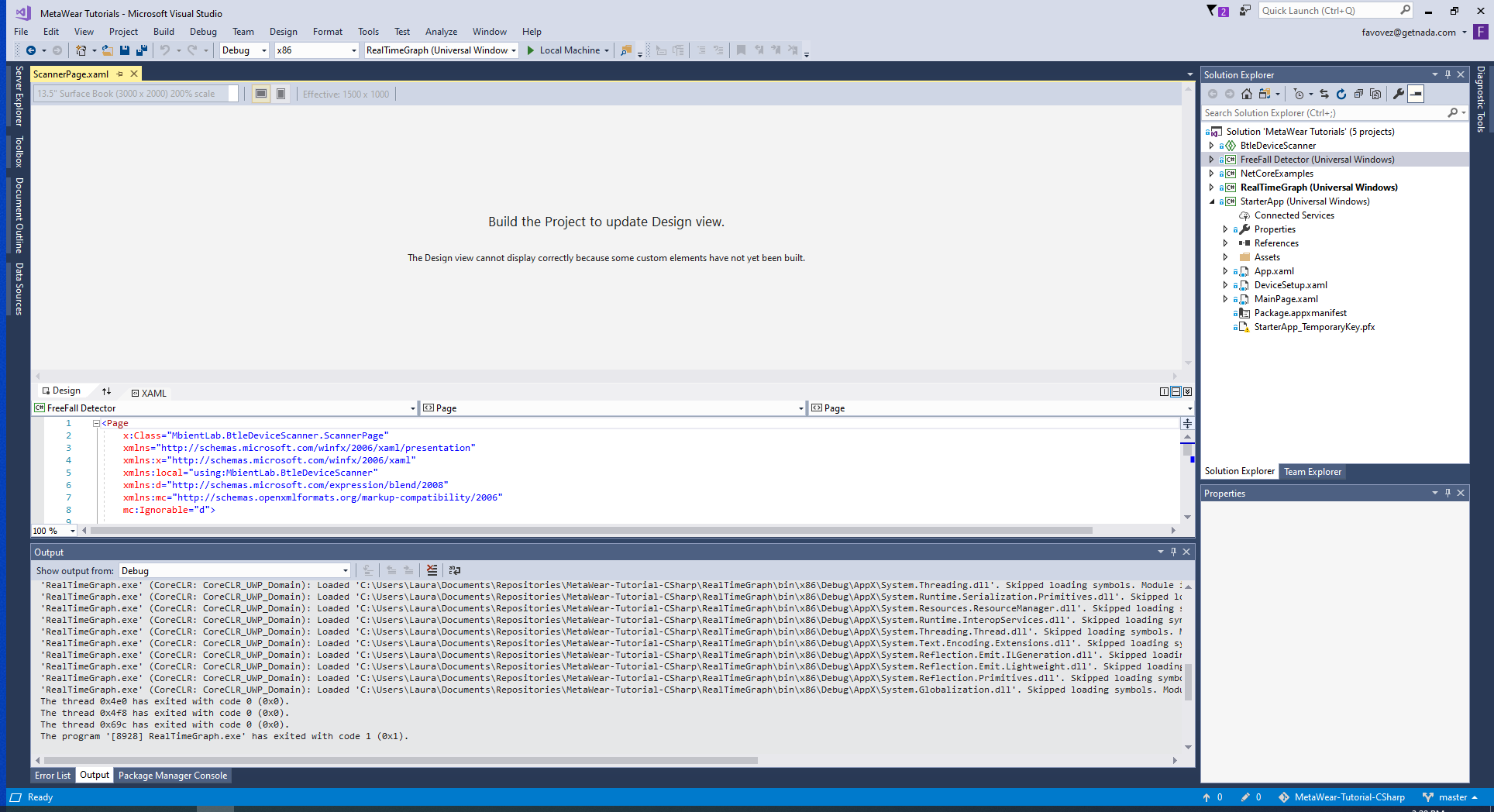
- Get your MetaWear board handy
- Run it!
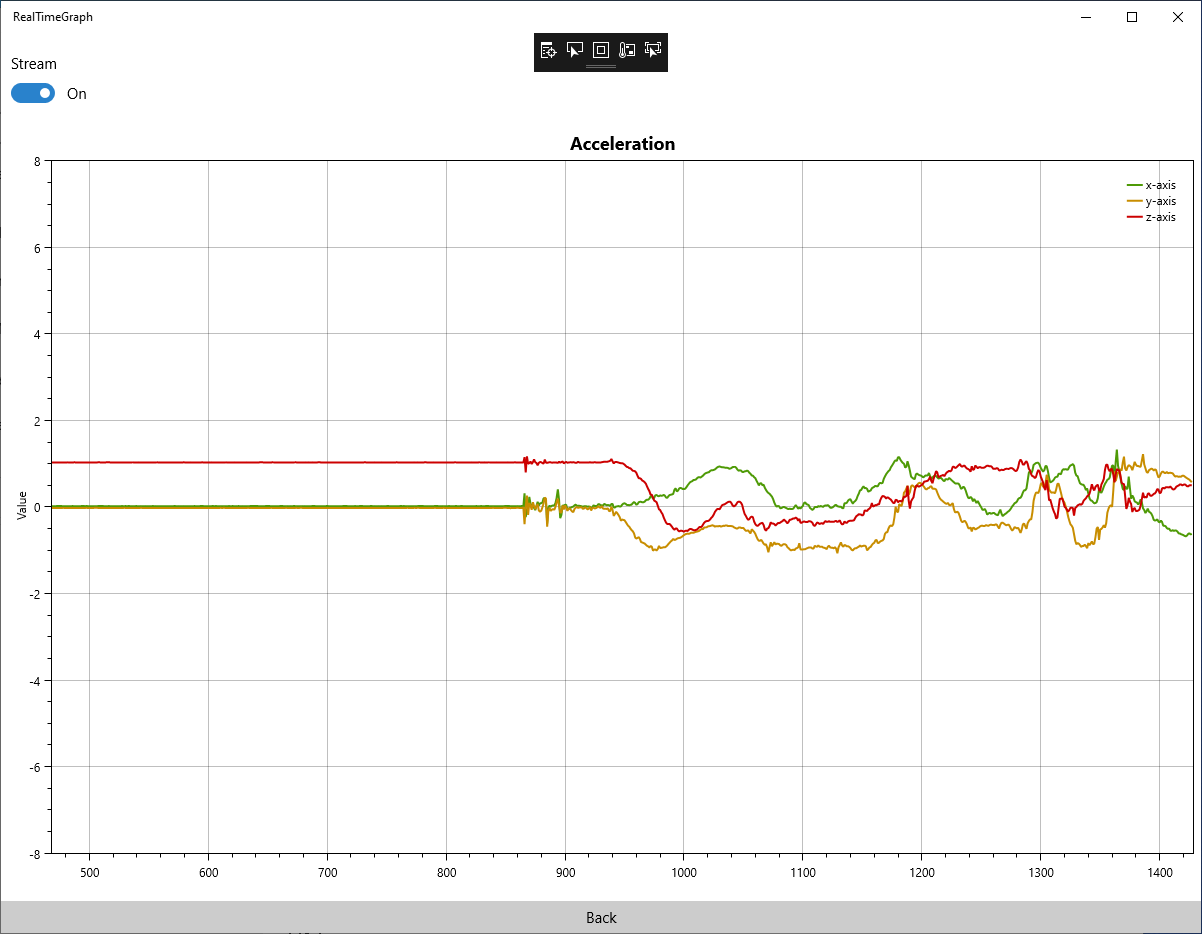
This will create a Windows App that can connet to a MetaWear board and then display a real time graph of the acceleration once you turn on the stream button.
You can try to play with the FreeFall Detector App as well:
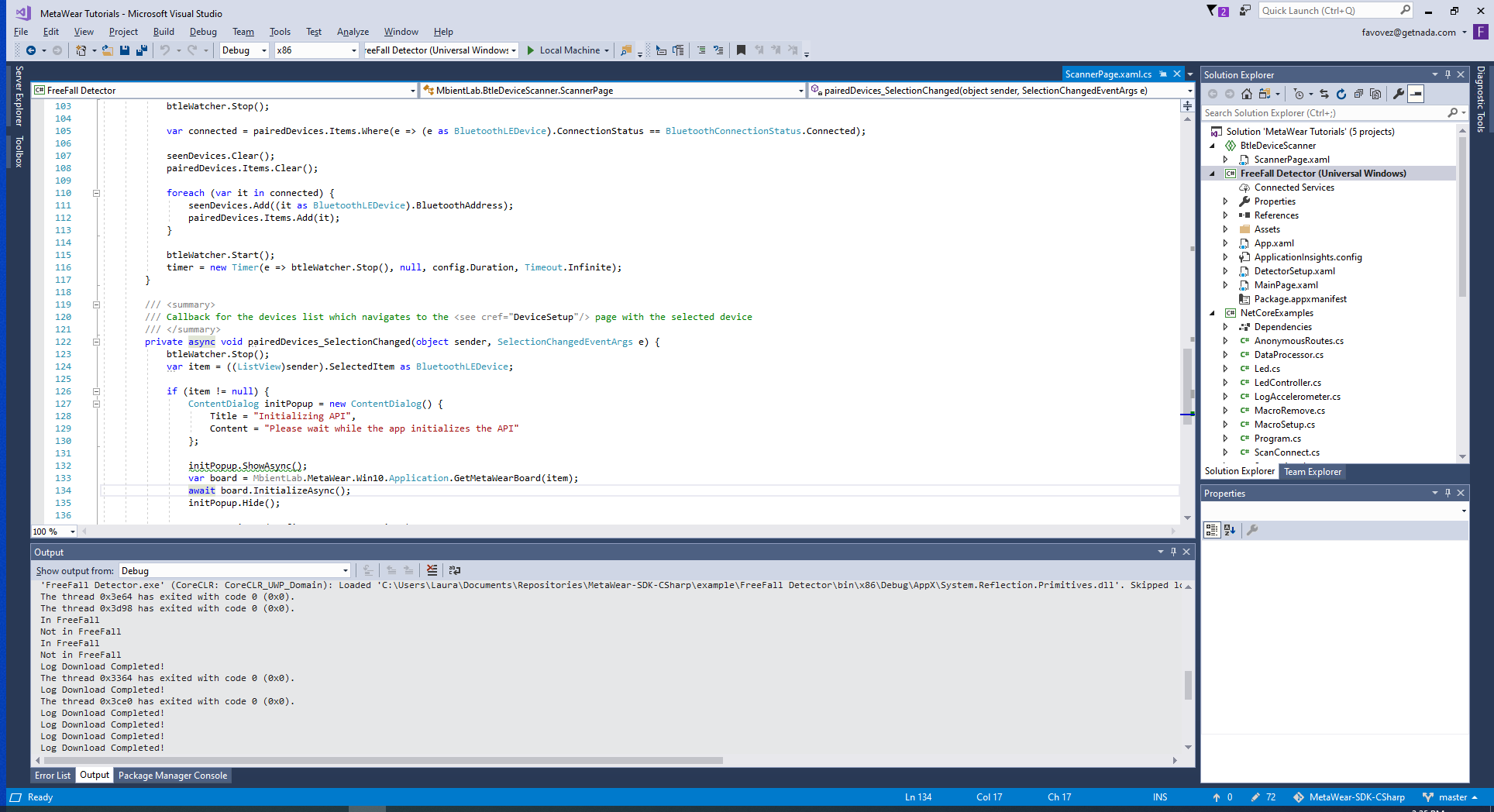
1.6. First App¶
A finished C# project with example code is located in the NetCoreExamples
folder.
1.7. Next Steps¶
Go over to our C# Developers Section to learn more about C# development.